Pocket Note has the function adding photos as below picture and I write how to get photos this time.
環境[Environment]:Xcode 8、 Swift 3
まず事前準備として、info.plistにCamera、Photo Libraryへのアクセス設定を行います。
NSPhotoLibraryUsageDescriptionがPhoto Libraryへアクセスする目的を記載するキーで、NSCameraUsageDescriptionがCameraにアクセスする目的を記載するキーです。
At first,you must open info.plist and set access privilege control of photo library and camera.NSPhotoLibraryUsageDescription is key for setting purpose of accessing photo library.NSCameraUsageDescription is key for setting purpose of accessing camera.
At first,you must open info.plist and set access privilege control of photo library and camera.NSPhotoLibraryUsageDescription is key for setting purpose of accessing photo library.NSCameraUsageDescription is key for setting purpose of accessing camera.
それではコードを書いてみます。
Then let's write code.
Then let's write code.
import UIKit
class ViewController: UIViewController,UIImagePickerControllerDelegate,UINavigationControllerDelegate {
let buttonCamera:UIButton = UIButton()
let buttonSavedPhotoAlbum:UIButton = UIButton()
let buttonPhotoLibarary:UIButton = UIButton()
let imageView:UIImageView = UIImageView()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
buttonCamera.frame = CGRect(x: 10, y: 20, width: 200, height: 50)
buttonCamera.layer.borderColor = UIColor.blue.cgColor
buttonCamera.layer.borderWidth = 1
buttonCamera.setTitle("Camera", for: .normal)
buttonCamera.setTitleColor(UIColor.blue, for: .normal)
buttonCamera.addTarget(self, action: #selector(self.touchUpButtonCamera), for: .touchUpInside)
self.view.addSubview(buttonCamera)
buttonSavedPhotoAlbum.frame = CGRect(x: 10, y: 80, width: 200, height: 50)
buttonSavedPhotoAlbum.layer.borderColor = UIColor.blue.cgColor
buttonSavedPhotoAlbum.layer.borderWidth = 1
buttonSavedPhotoAlbum.setTitle("SavedPhotoAlbum", for: .normal)
buttonSavedPhotoAlbum.setTitleColor(UIColor.blue, for: .normal)
buttonSavedPhotoAlbum.addTarget(self, action: #selector(self.touchUpButtonSavedPhotoAlbum), for: .touchUpInside)
self.view.addSubview(buttonSavedPhotoAlbum)
buttonPhotoLibarary.frame = CGRect(x: 10, y: 140, width: 200, height: 50)
buttonPhotoLibarary.layer.borderColor = UIColor.blue.cgColor
buttonPhotoLibarary.layer.borderWidth = 1
buttonPhotoLibarary.setTitle("PhotoLibrary", for: .normal)
buttonPhotoLibarary.setTitleColor(UIColor.blue, for: .normal)
buttonPhotoLibarary.addTarget(self, action: #selector(self.touchUpButtonPhotoLibrary), for: .touchUpInside)
self.view.addSubview(buttonPhotoLibarary)
imageView.frame = CGRect(x: 10, y: 200, width: 500, height: 500)
self.view.addSubview(imageView)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func touchUpButtonCamera(){
showPicker(sourceType: UIImagePickerControllerSourceType.camera)
}
func touchUpButtonSavedPhotoAlbum(){
showPicker(sourceType: UIImagePickerControllerSourceType.savedPhotosAlbum)
}
func touchUpButtonPhotoLibrary(){
showPicker(sourceType: UIImagePickerControllerSourceType.photoLibrary)
}
//画像選択画面 or カメラ 表示(Show Photo Album or Camera)
func showPicker(sourceType:UIImagePickerControllerSourceType){
//1.Camera、Photo Albumへのアクセス権を取得(Get access privilege control of camera or photo album)
if !UIImagePickerController.isSourceTypeAvailable(sourceType){
return
}
//2.ImagePickerを表示(Show ImagePicker)
let picker = UIImagePickerController()
picker.sourceType = sourceType
picker.delegate = self
self.present(picker,animated:true,completion:nil)
}
//画像選択後の処理(Process after selecting photo)
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : AnyObject]){
//3.画面を閉じて、選択されたImageを取得(Close screen and get selected image)
picker.presentingViewController?.dismiss(animated: true, completion:nil)
var image:UIImage! = info[UIImagePickerControllerOriginalImage] as! UIImage
//4.Cameraで写真を撮った場合はカメラロールに保存(If camera is choosen,save image to Camera Roll)
if (picker.sourceType == UIImagePickerControllerSourceType.camera){
UIImageWriteToSavedPhotosAlbum(image, self, nil, nil)
}
//5.imageのサイズを縮小(Reduce an image)
let widthValue:CGFloat = image.size.width
let heightValue:CGFloat = image.size.height
let scaleValue:CGFloat = 500/widthValue
let size = CGSize(width: widthValue*scaleValue, height: heightValue*scaleValue)
UIGraphicsBeginImageContext(size)
image.draw(in: CGRect(x:0, y:0, width:size.width, height:size.height))
var resizeImage:UIImage! = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
image = nil
imageView.image = resizeImage
imageView.contentMode = UIViewContentMode.scaleAspectFit
resizeImage = nil
}
//画像選択キャンセル後の処理(Cancel ImagePikerContoller)
func imagePickerControllerDidCancel(_ picker: UIImagePickerController) {
picker.presentingViewController?.dismiss(animated: true,completion:nil)
}
}
Camera、PhotoLibraryにアクセスするにはUIImagePickerを使用します。どこにアクセスするかはUIImagePickerControllerSourceTypeで指定します。
Use UIImagePicker for access Camera and PhotoLibrary and use UIImagePickerControllerSourceType to specify access type.
UIImagePickerControllerSourceType.Camera :カメラにアクセス(Access Camera)
UIImagePickerControllerSourceType.PhotoLibrary : フォトアルバムにアクセス(Access Photo Library)
UIImagePickerControllerSourceType.SavedPhotoAlbum:フォトアルバムのモーメントにアクセス(Access Moment of Photo Library)
またUIImagePickerのイベントを受け取る為にUIImagePickerControllerDelegate、UINavigationControllerDelegateを実装します。
And implement UIImagePickerControllerDelegate and UINavigationControllerDelegate for receiving UIIMagePicker's events.
Camera、Photo Libraryが使用できるかどうかをisSourceTypeAvailableで確認します。この時、以下のメッセージが表示され、info.plistに設定した目的も表示されます。
Check that Camera and Photo Library can be used using isSourceTypeAvailable.And this message is displayed with purpose of info.plist.
画像が選択されるとdidFinishPickingMediaInfoでImageが渡されます。Cameraを選択した時はUIImageWriteToSavedPhotoAlbumでPhoto Libraryのカメラロールに保存します。
また読み込んだ画像のサイズは非常に大きい為、アプリで必要なサイズに縮小しておきます。
When image is selected,get image using didFinishPickingMediaInfo.
If Camera is selected,save image to Camera Roll of Photo Library using UIImageWriteToSavedPhotoAlbum.
Size of image is suppressed required for an application in this sample code,because original image size is big.

にほんブログ村
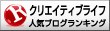
クリエイティブライフ ブログランキングへ
0 件のコメント:
コメントを投稿